Table of Contents
1. Introduction to Deep Learning
1.1 What is Deep Learning?
Deep Learning is a subset of machine learning that deals with algorithms inspired by the structure and function of the brain, known as neural networks. The term “deep” refers to the number of layers in the network, which can enable the model to learn complex patterns in large datasets.
Historically, deep learning gained traction in the early 2010s, thanks largely to advances in computational power and the availability of large datasets. Unlike machine learning, which usually requires hand-crafted features, deep learning can automatically learn features from raw data.
In the real world, deep learning is making waves in various areas, such as:
- Image recognition
- Natural language processing
- Autonomous vehicles
- Medical diagnostics
1.2 Importance of Python in Deep Learning
Python is a go-to programming language in the field of deep learning, thanks to its simplicity and clarity. It boasts several powerful libraries and frameworks like:
- TensorFlow: Developed by Google, great for production-level models.
- Keras: High-level API for TensorFlow, ideal for beginners.
- PyTorch: Instagram’s favorite for dynamic computation graphs, particularly strong in research settings.
Using Python brings numerous advantages, including:
- A beginner-friendly syntax, making it easier for newcomers.
- A vast ecosystem of libraries tailored for data analysis and visualization.
- A supportive community offering countless resources, tutorials, and forums for learning and troubleshooting.
1.3 Overview of the Tutorial
This tutorial aims to guide you through the entire journey of deep learning using Python. By the end, you should feel comfortable building your own models from scratch.
Objectives and goals of the tutorial:
- Understand the core concepts of deep learning.
- Set up your environment for deep learning tasks.
- Implement various types of neural networks.
Prerequisites for readers:
- Basic understanding of Python programming.
- Familiarity with fundamental concepts in machine learning is helpful but not required.
Structure and content overview:
The tutorial is split into several sections covering everything from setting up your environment to implementing complex models. Let’s dive in!
2. Setting Up Your Environment
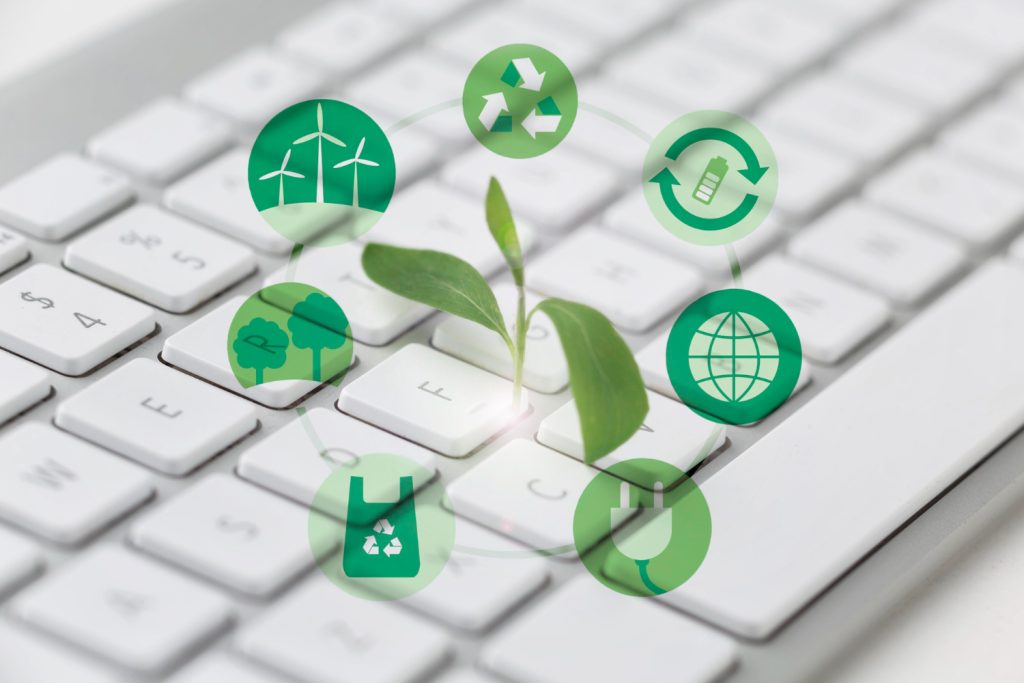
2.1 Installing Python and Necessary Libraries
To get started, you need Python installed on your machine. Follow these detailed steps:
- Download Python: Go to the Python official website and download the latest version suitable for your operating system.
- Install Python: During installation, make sure to check the box that says “Add Python to PATH.”
- Set up a virtual environment:
Use the following commands in your terminal:python -m venv myenv source myenv/bin/activate # for macOS/Linux
myenv\Scripts\activate # for Windows
- Installing essential libraries: Use pip to install libraries essential for data manipulation and numerical computing:
pip install numpy pandas matplotlib
2.2 Choosing an Integrated Development Environment (IDE)
An IDE can make your programming experience smoother. Here are a few popular options:
- Jupyter Notebook: Great for interactive coding and data visualization. Ideal for beginners.
- PyCharm: Feature-rich, good for larger projects. It has a steeper learning curve.
- Google Colab: Free and cloud-based, perfect for GPU access without local setup.
Consider your needs and preferences when choosing an IDE. For example, if you prefer working in a web browser, Google Colab might be your best bet.
2.3 Working with GPU Acceleration
Using a Graphics Processing Unit (GPU) can significantly speed up your deep learning tasks. Here’s how to set it up:
- Importance of GPU: GPUs handle parallel processing, making them suitable for the computations needed in training deep learning models.
- Install CUDA and cuDNN: This can vary based on your system, but generally, you can download them from NVIDIA’s official site and follow their installation instructions.
- Configuring your environment: Ensure that Python can access these libraries by setting up environment variables or installing specific versions based on your GPU drivers.
3. Core Concepts of Deep Learning
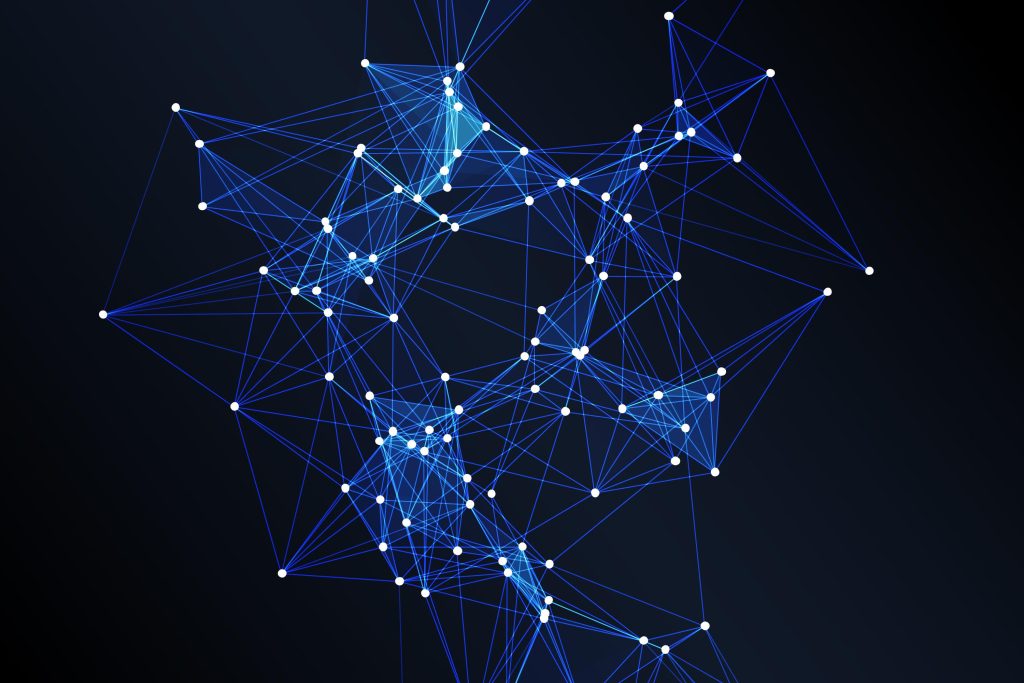
3.1 Neural Networks Explained
At its core, a neural network is made up of:
- Neurons: The basic units that process input and pass it to the next layer.
- Layers: Composed of neurons, including:
- Input layer: Receives the input.
- Hidden layers: Process the inputs from the previous layer.
- Output layer: Produces the output.
Different types of neural networks serve different purposes:
- Fully Connected Networks: Commonly used for simple tasks.
- Convolutional Neural Networks (CNNs): Best suited for image-related tasks.
- Recurrent Neural Networks (RNNs): Ideal for sequence data like time series and natural language.
Activation functions like ReLU and sigmoid help introduce non-linearity into the network, allowing it to tackle complex problems.
3.2 Training Neural Networks
Training a neural network involves teaching it to minimize the difference between its predictions and actual outcomes. Here’s an overview:
- Loss functions: Quantify how well the model’s predictions match the actual data. Common examples include Mean Squared Error for regression and Cross-Entropy for classification.
- Optimizers: Such as Adam or SGD, adjust the weights of the neurons based on the gradients calculated from the loss function.
Gradient descent and backpropagation are the backbone of neural network training, helping to adjust the model in the right direction.
3.3 Evaluating Model Performance
To measure how well your model performs, you should look at:
- Accuracy, precision, and recall: Important metrics that indicate the model’s performance.
- Validation and testing: Use separate data to validate the model’s ability to generalize to unseen data.
- Strategies for improvement: Techniques such as regularization can help prevent overfitting, and hyperparameter tuning can help find the best model configuration.
4. Implementing Deep Learning Models
4.1 Building Your First Neural Network
Let’s create a simple neural network using Keras. Here’s a step-by-step guide:
- Data preprocessing: Load your dataset (e.g., the MNIST dataset of handwritten digits). Normalize the data for better performance.
- Create the model:
from keras.models import Sequential
from keras.layers import Dense
model = Sequential()
model.add(Dense(64, activation='relu', input_shape=(input_dim,))) model.add(Dense(10, activation='softmax'))
- Compile the model:
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
- Fit the model:
model.fit(X_train, y_train, epochs=10, batch_size=32)
4.2 Convolutional Neural Networks (CNNs)
CNNs are particularly effective for tasks involving image data. Here are some key components:
- Convolutional layers: Use filters to capture features like edges or textures.
- Pooling layers: Reduce dimensions while retaining important information.
- Fully connected layers: Connect the flattened output to the final output layer.
To build a CNN for image classification, you can use the following structure:
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(image_height, image_width, channels)))
model.add(MaxPooling2D((2, 2)))
model.add(Flatten())
model.add(Dense(64, activation='relu'))
model.add(Dense(num_classes, activation='softmax'))
4.3 Recurrent Neural Networks (RNNs)
RNNs shine when dealing with sequence data like text or time series. They can remember previous inputs, thanks to their loop structures.
Types of RNNs include:
- LSTM: Long Short-Term Memory, designed to overcome the vanishing gradient problem.
- GRU: Gated Recurrent Unit, a simpler version of LSTMs.
Implementing a basic RNN for natural language processing can look like this:
from keras.models import Sequential
from keras.layers import LSTM, Dense
model = Sequential()
model.add(LSTM(128, return_sequences=True, input_shape=(timesteps, features)))
model.add(LSTM(128))
model.add(Dense(num_classes, activation='softmax'))
5. Advanced Topics in Deep Learning
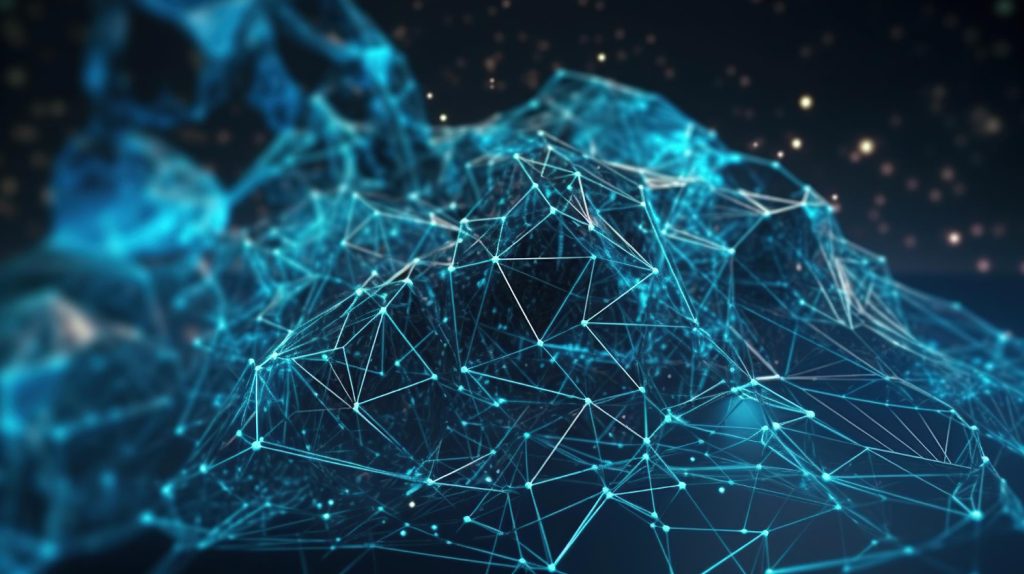
5.1 Transfer Learning
Transfer learning involves taking a pre-trained model and fine-tuning it for a new task. This is beneficial, especially when you have limited data for a specific task.
You would typically:
1. Load a pre-trained model (like VGG16 or ResNet).
2. Freeze the base layers.
3. Add custom layers on top for your specific task.
5.2 Generative Adversarial Networks (GANs)
GANs consist of two neural networks, a generator and a discriminator, that compete against each other. Here’s a brief overview of how they function:
+ The generator tries to create realistic data (e.g., images).
+ The discriminator attempts to distinguish between real and generated data.
Implementing a simple GAN can be a bit complex but rewarding.
5.3 Ethics and Considerations in Deep Learning
As deep learning continues to evolve, ethical considerations become increasingly important:
+ Bias and fairness: It’s crucial to ensure that your models do not propagate or amplify societal biases.
+ Explainability: Users should be able to understand how and why decisions are made by AI systems.
Some organizations implement guidelines to ensure their AI products are ethical and equitable.
Conclusion
In this comprehensive tutorial, we explored the wide world of deep learning and how Python plays a key role in its implementation. From foundational concepts to advanced topics, you should now feel equipped to embark on your deep learning journey.
As you continue, remember that practical experience is invaluable. Start working on projects, contribute to open-source repositories, and stay curious. There’s a vast ocean of resources out there waiting for you to dive in. Keep exploring!
FAQs
Q. What programming skills do I need to start learning deep learning with Python?
A basic understanding of Python is essential, along with familiarity with data structures and programming concepts.
Q. How long does it take to become proficient in deep learning?
It varies, but with consistent practice and study, many people become proficient in several months to a year.
Q. What are some recommended projects to practice deep learning skills?
Consider projects like image classification, sentiment analysis, or even building a simple chatbot.
Q. Can I use deep learning for non-technical fields?
Absolutely! Deep learning can be applied to various fields, from healthcare to finance and even education.
Q. How can I stay updated with the latest trends in deep learning?
Follow blogs, subscribe to newsletters, and join online communities like Reddit and Stack Overflow for continuous learning and networking.